In Python, variables are used to store data that can be referenced and manipulated during program execution. A variable is essentially a name that is assigned to a value. Unlike many other programming languages, Python variables do not require explicit declaration of type. The type of the variable is inferred based on the value assigned.
In this article, we’ll explore the concept of variables in Python, including their syntax, characteristics, and common operations.
What are variables in Python?
Variables act as placeholders for data. They allow us to store and reuse values in our program.
Example:
Python
# Variable 'x' stores the integer value 10
x = 5
# Variable 'name' stores the string "Samantha"
name = "Samantha"
Rules for Naming Variables
To use variables effectively, we must follow Python’s naming rules:
- Variable names can only contain letters, digits and underscores (
_
). - A variable name cannot start with a digit.
- Variable names are case-sensitive (
myVar
and myvar
are different). - Avoid using Python keywords (e.g.,
if
, else
, for
) as variable names.
Valid Example:
Python
age = 21
_colour = "lilac"
total_score = 90
Invalid Example:
Python
1name = "Error" # Starts with a digit
class = 10 # 'class' is a reserved keyword
user-name = "Doe" # Contains a hyphen
Assigning Values to Variables
Basic Assignment
Variables in Python are assigned values using the =
operator.
Python
Dynamic Typing
Python variables are dynamically typed, meaning the same variable can hold different types of values during execution.
Python
x = 10
x = "Now a string"
Multiple Assignments
Python allows multiple variables to be assigned values in a single line.
Assigning the Same Value
Python allows assigning the same value to multiple variables in a single line, which can be useful for initializing variables with the same value.
Python
a = b = c = 100
print(a, b, c)
Assigning Different Values
We can assign different values to multiple variables simultaneously, making the code concise and easier to read.
Python
x, y, z = 1, 2.5, "Python"
print(x, y, z)
Casting a Variable
Casting refers to the process of converting the value of one data type into another. Python provides several built-in functions to facilitate casting, including int(), float() and str() among others.
Basic Casting Functions
- int() – Converts compatible values to an integer.
- float() – Transforms values into floating-point numbers.
- str() – Converts any data type into a string.
Examples of Casting:
Python
# Casting variables
s = "10" # Initially a string
n = int(s) # Cast string to integer
cnt = 5
f = float(cnt) # Cast integer to float
age = 25
s2 = str(age) # Cast integer to string
# Display results
print(n)
print(cnt)
print(s2)
Getting the Type of Variable
In Python, we can determine the type of a variable using the type() function. This built-in function returns the type of the object passed to it.
Example Usage of type()
Python
# Define variables with different data types
n = 42
f = 3.14
s = "Hello, World!"
li = [1, 2, 3]
d = {'key': 'value'}
bool = True
# Get and print the type of each variable
print(type(n))
print(type(f))
print(type(s))
print(type(li))
print(type(d))
print(type(bool))
Output<class 'int'>
<class 'float'>
<class 'str'>
<class 'list'>
<class 'dict'>
<class 'bool'>
Scope of a Variable
There are two methods how we define scope of a variable in python which are local and global.
Local Variables:
Variables defined inside a function are local to that function.
Python
def f():
a = "I am local"
print(a)
f()
# print(a) # This would raise an error since 'local_var' is not accessible outside the function
Global Variables:
Variables defined outside any function are global and can be accessed inside functions using the global
keyword.
Python
a = "I am global"
def f():
global a
a = "Modified globally"
print(a)
f()
print(a)
OutputModified globally
Modified globally
Object Reference in Python
Let us assign a variable x to value 5.
x = 5

When x = 5
is executed, Python creates an object to represent the value 5
and makes x
reference this object.
Now, if we assign another variable y to the variable x.
y = x
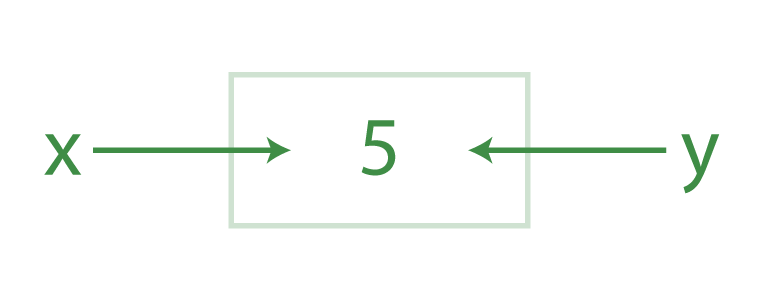
Explanation:
- Python encounters the first statement, it creates an object for the value
5
and makes x
reference it. The second statement creates y
and references the same object as x
, not x
itself. This is called a Shared Reference, where multiple variables reference the same object.
Now, if we write
x = 'Geeks'
Python creates a new object for the value "Geeks"
and makes x
reference this new object.
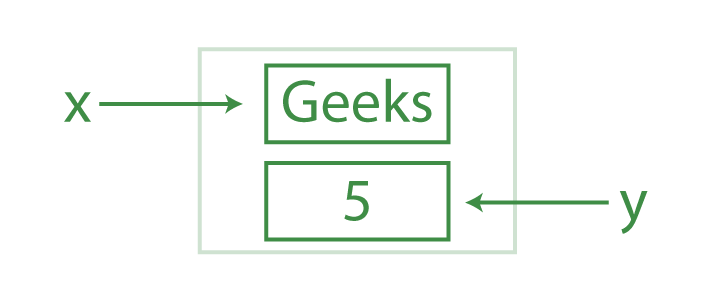
Explanation:
- The variable
y
remains unchanged, still referencing the original object 5
.
If we now assign a new value to y
:
y = "Computer"

- Python creates yet another object for
"Computer"
and updates y
to reference it. - The original object
5
no longer has any references and becomes eligible for garbage collection.
Key Takeaways:
- Python variables hold references to objects, not the actual objects themselves.
- Reassigning a variable does not affect other variables referencing the same object unless explicitly updated.
Delete a Variable Using del
Keyword
We can remove a variable from the namespace using the del
keyword. This effectively deletes the variable and frees up the memory it was using.
Example:
Python
# Assigning value to variable
x = 10
print(x)
# Removing the variable using del
del x
# Trying to print x after deletion will raise an error
# print(x) # Uncommenting this line will raise NameError: name 'x' is not defined
Explanation:
del x
removes the variable x
from memory.- After deletion, trying to access the variable
x
results in a NameError
, indicating that the variable no longer exists.
Practical Examples
1. Swapping Two Variables
Using multiple assignments, we can swap the values of two variables without needing a temporary variable.
Python
a, b = 5, 10
a, b = b, a
print(a, b)
2. Counting Characters in a String
Assign the results of multiple operations on a string to variables in one line.
Python
word = "Python"
length = len(word)
print("Length of the word:", length)
OutputLength of the word: 6
Frequently Asked Questions(FAQ’s) on Python Variables
What is the scope of a variable in Python?
The scope of a variable determines where it can be accessed. Local variables are scoped to the function in which they are defined, while global variables can be accessed throughout the program.
Can we change the type of a variable after assigning it?
Yes, Python allows dynamic typing. A variable can hold a value of one type initially and be reassigned a value of a different type later.
What happens if we use an undefined variable?
Using an undefined variable raises a NameError
. Always initialize variables before use.
How can we delete a variable in Python?
We can delete a variable in Python using the del
keyword:
x = 10
del x
#print(x) # Raises a NameError since 'x' has been deleted