Python For Loops are used for iterating over a sequence like lists, tuples, strings, and ranges.
- For loop allows you to apply the same operation to every item within loop.
- Using For Loop avoid the need of manually managing the index.
- For loop can iterate over any iterable object, such as dictionary, list or any custom iterators.
For Loop Example:
Python
s = ["Geeks", "for", "Geeks"]
# using for loop with string
for i in s:
print(i)
Flowchart of Python For Loop
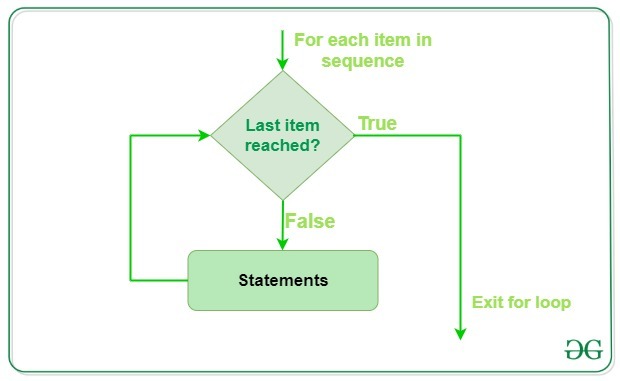
For Loop flowchart
Python For Loop Syntax
for var in iterable:
# statements
pass
Note: In Python, for loops only implement the collection-based iteration.
Python For Loop with String
This code uses a for loop to iterate over a string and print each character on a new line. The loop assigns each character to the variable i and continues until all characters in the string have been processed.
Python
s = "Geeks"
for i in s:
print(i)
Using range() with For Loop
The range() function is commonly used with for loops to generate a sequence of numbers. It can take one, two, or three arguments:
- range(stop): Generates numbers from 0 to stop-1.
- range(start, stop): Generates numbers from start to stop-1.
- range(start, stop, step): Generates numbers from start to stop-1, incrementing by step.
Python
for i in range(0, 10, 2):
print(i)
Control Statements with For Loop
Loop control statements change execution from their normal sequence. When execution leaves a scope, all automatic objects that were created in that scope are destroyed. Python supports the following control statements.
Continue with For Loop
Python continue Statement returns the control to the beginning of the loop.
Python
# Prints all letters except 'e' and 's'
for i in 'geeksforgeeks':
if i == 'e' or i == 's':
continue
print(i)
Break with For Loop
Python break statement brings control out of the loop.
Python
for i in 'geeksforgeeks':
# break the loop as soon it sees 'e'
# or 's'
if i == 'e' or i == 's':
break
print(i)
Pass Statement with For Loop
The pass statement to write empty loops. Pass is also used for empty control statements, functions, and classes.
Python
# An empty loop
for i in 'geeksforgeeks':
pass
print(i)
Else Statement with For Loops
Python also allows us to use the else condition for loops. The else block just after for/while is executed only when the loop is NOT terminated by a break statement.
Python
for i in range(1, 4):
print(i)
else: # Executed because no break in for
print("No Break\n")
Using Enumerate with for loop
In Python, enumerate() function is used with the for loop to iterate over an iterable while also keeping track of index of each item.
Python
li = ["eat", "sleep", "repeat"]
for i, j in enumerate(li):
print (i, j)
Output0 eat
1 sleep
2 repeat
Nested For Loops in Python
This code uses nested for loops to iterate over two ranges of numbers (1 to 3 inclusive) and prints the value of i and j for each combination of these two loops. \
The inner loop is executed for each value of i in outer loop. The output of this code will print the numbers from 1 to 3 three times, as each value of i is combined with each value of j.
Python
for i in range(1, 4):
for j in range(1, 4):
print(i, j)
Output1 1
1 2
1 3
2 1
2 2
2 3
3 1
3 2
3 3
Python For Loop Exercise Questions
Below are two Exercise Questions on Python for-loops. We have covered continue statement and range() function in these exercise questions.
Q1. Code to implement Continue statement in for-loop
Python
a = ["shirt", "sock", "pants", "sock", "towel"]
b = []
for i in a:
if i == "sock":
continue
else:
print(f"Washing {i}")
b.append("socks")
print(f"Washing {b}")
OutputWashing shirt
Washing pants
Washing towel
Washing ['socks']
Q2. Code to implement range function in for-loop
Python
for i in range(1, 8):
d = 3 + (i - 1) * 0.5
print(f"Day {i}: Run {d:.1f} miles")
OutputDay 1: Run 3.0 miles
Day 2: Run 3.5 miles
Day 3: Run 4.0 miles
Day 4: Run 4.5 miles
Day 5: Run 5.0 miles
Day 6: Run 5.5 miles
Day 7: Run 6.0 miles
Test Your Knowledge – Python For Loop Quiz
Python For Loops – FAQs
What is syntax of for loop in Python?
The syntax of a for loop in Python is straightforward. It iterates over a sequence (like a list, tuple, string, etc.) and executes the block of code inside the loop for each element in the sequence.
for item in sequence:
# Code block to execute
How to iterate with an index in a for loop in Python?
You can use the ‘enumerate()’ function to iterate over a sequence and retrieve both the index and the value of each element.
for index, item in enumerate(sequence):
# Use index and item inside the loop
Can you provide examples of for loops in Python?
Sure! Here are some examples of for loops in Python:
# Example 1: Iterating over a list
fruits = ['apple', 'banana', 'cherry']
for fruit in fruits:
print(fruit)
# Example 2: Iterating over a string
for char in 'Python':
print(char)
# Example 3: Using enumerate to get index and value
for index, num in enumerate([10, 20, 30]):
print(f'Index {index}: {num}')
# Example 4: Iterating over a dictionary
person = {'name': 'John', 'age': 30}
for key, value in person.items():
print(f'{key}: {value}')
How to write a for loop in Python?
To write a for loop, specify the variable that will hold each item from the sequence (‘item’ in the examples above), followed by the keyword in and the sequence itself (‘sequence’ in the examples).
# Basic syntax
for item in sequence:
# Code block to execute
How to use for loops in Python?
For loops are used to iterate over sequences (like lists, tuples, strings, dictionaries) and perform operations on each element or key-value pair. They are fundamental for iterating and processing data in Python.
# Example: Calculating sum of numbers in a list
numbers = [1, 2, 3, 4, 5]
total = 0
for num in numbers:
total += num
print(f'Total sum: {total}')